Building an Employee Management System with .NET Core Web API
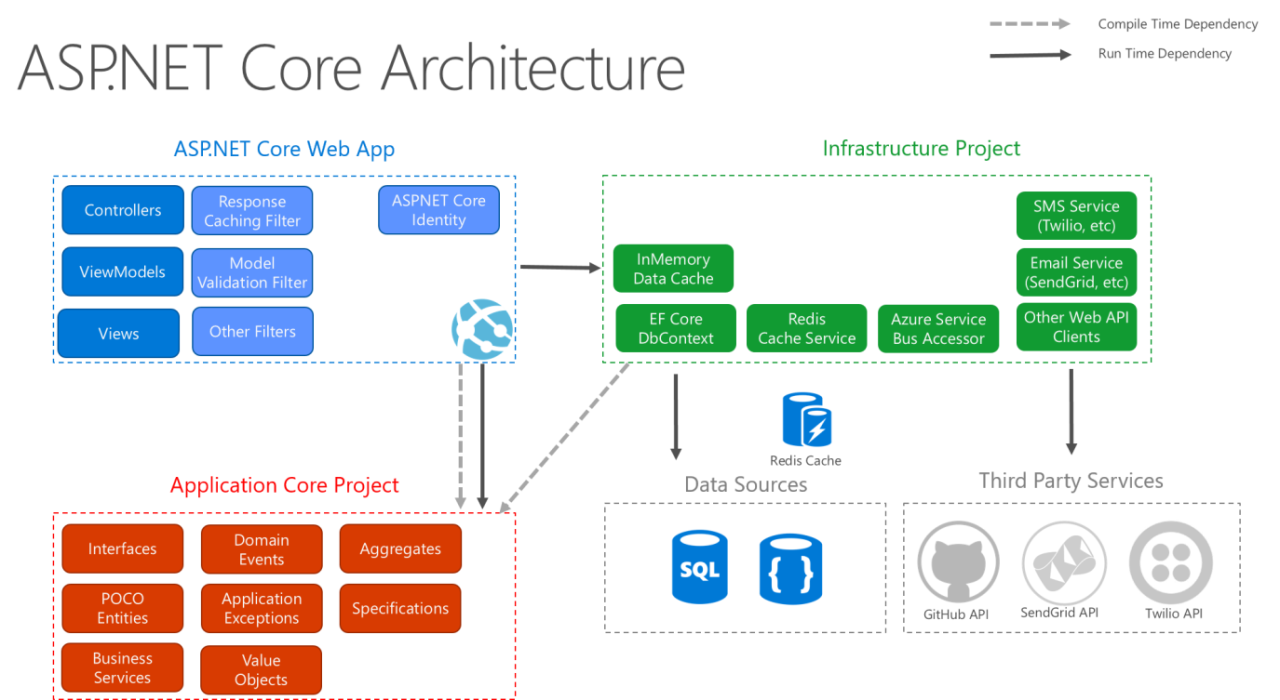
Introduction
Managing employee data efficiently is crucial for any organization. To help our client improve HR operations, we developed a scalable and secure Employee Management System using .NET Core Web API. This blog highlights the use of .NET Core Web API to create a real-time, efficient backend system that integrates seamlessly with other enterprise applications.
Client Overview and Challenges
Client Overview
Our client is a growing IT company with over 5,000 employees spread across multiple global offices. The HR department faced challenges in managing employee data, onboarding, and tracking performance metrics.
Challenges
- Inefficient Data Management: Employee records were stored across multiple systems, making it hard to retrieve or update information.
- Manual Onboarding: The onboarding process involved excessive paperwork and lacked automation.
- Performance Tracking Issues: Managers had no centralized way to track and evaluate employee performance.
- Limited Integration: Existing systems could not integrate with payroll, benefits, or project management tools.
Solution: Employee Management System
We developed an Employee Management System backend using .NET Core Web API. The system was designed to address the client’s pain points by offering a RESTful API to manage employee data and integrate with other enterprise tools.
Key Features Implemented
1. RESTful API for CRUD Operations
- Problem: Employee data was scattered across multiple systems.
- Solution:
- Developed RESTful endpoints for creating, retrieving, updating, and deleting (CRUD) employee data.
- Key API endpoints:
- GET /employees: Retrieve all employees.
- GET /employees/{id}: Retrieve a specific employee by ID.
- POST /employees: Add a new employee.
- PUT /employees/{id}: Update employee details.
- DELETE /employees/{id}: Remove an employee record.
Outcome:
- Centralized employee data for easier access and management.
2. Role-Based Access Control (RBAC)
- Problem: Sensitive employee data needed to be secured.
- Solution:
- Implemented JWT (JSON Web Token) authentication.
- Defined role-based access controls:
- Admin: Full access to all API endpoints.
- Manager: Access to employee data within their team.
- Employee: Access to their own records.
Outcome:
- Enhanced data security and compliance with data protection regulations.
3. Employee Onboarding Automation
- Problem: The onboarding process was manual and time-consuming.
- Solution:
- Created an onboarding workflow using Azure Logic Apps.
- Integrated with the .NET Core Web API to:
- Automatically generate employee IDs.
- Send onboarding emails with necessary documents and links.
- Notify HR and IT teams to set up accounts and equipment.
Outcome:
- Reduced onboarding time by 50%, improving new hire experience.
4. Real-Time Notifications
- Problem: Employees and managers lacked updates on critical events.
- Solution:
- Integrated SignalR with the .NET Core Web API for real-time notifications.
- Examples of notifications:
- Employee onboarding status.
- Performance review reminders.
- Changes to employee records.
Outcome:
- Improved communication and efficiency across departments.
5. Performance Tracking and Reporting
- Problem: No centralized system for tracking employee performance.
- Solution:
- Added API endpoints for submitting and retrieving performance reviews.
- Integrated with Power BI to provide visual performance dashboards.
- Key performance metrics:
- Attendance records.
- Project contributions.
- Feedback from managers and peers.
Outcome:
- Managers gained actionable insights to improve team performance.
6. Integration with Payroll and Benefits
- Problem: Disconnected systems for payroll and benefits management.
- Solution:
- Exposed APIs to integrate with third-party tools like ADP and Workday.
- Automated data syncing for:
- Salary updates.
- Leave balances.
- Benefits enrollment.
Outcome:
- Reduced manual work and eliminated data inconsistencies.
Architecture and Tools
- Backend Framework: .NET Core Web API
- Database: SQL Server with Entity Framework Core for ORM.
- Authentication: JWT with role-based access control.
- Real-Time Communication: SignalR for notifications.
- Deployment: Hosted on Azure App Service with auto-scaling.
- Documentation: Used Swagger (OpenAPI) to document all API endpoints.
Implementation Process
- Requirement Analysis: Collaborated with the client’s HR and IT teams to identify requirements and pain points.
- API Development: Developed modular and reusable APIs following RESTful principles.
- Testing: Conducted unit, integration, and performance tests to ensure API reliability.
- Integration: Connected the API with third-party tools like ADP, Workday, and Power BI.
- Deployment: Deployed the API on Azure with CI/CD pipelines using Azure DevOps.
Results Achieved
- Efficiency Gains: Reduced HR operational workload by 40%.
- Faster Onboarding: Onboarding time decreased from 5 days to 2 days.
- Scalability: The system handled a 300% increase in API requests during peak hiring seasons.
- Improved Security: Achieved compliance with GDPR and other data protection standards.
- Data Insights: Enabled data-driven decision-making through performance dashboards.
Use Case: High-Volume Hiring Drive
Scenario:
The client initiated a hiring drive, onboarding 1,000 new employees in a single quarter.
Implementation:
- The .NET Core Web API auto-scaled on Azure to handle high API request volumes.
- Automated onboarding workflows ensured all new hires received credentials, equipment, and benefits enrollment within 48 hours.
Outcome:
- The client successfully onboarded employees without any delays or data errors.
Why Choose .NET Core Web API?
- Cross-Platform Compatibility: Develop once and deploy on Windows, Linux, or macOS.
- High Performance: Lightweight and optimized for large-scale applications.
- Ease of Integration: Seamlessly connect with third-party tools and services.
- Scalability: Handle growing workloads with Azure's flexible hosting solutions.
- Security: Built-in authentication and encryption tools ensure data safety.