Simplifying Insurance Claims Processing with .NET Core Web API
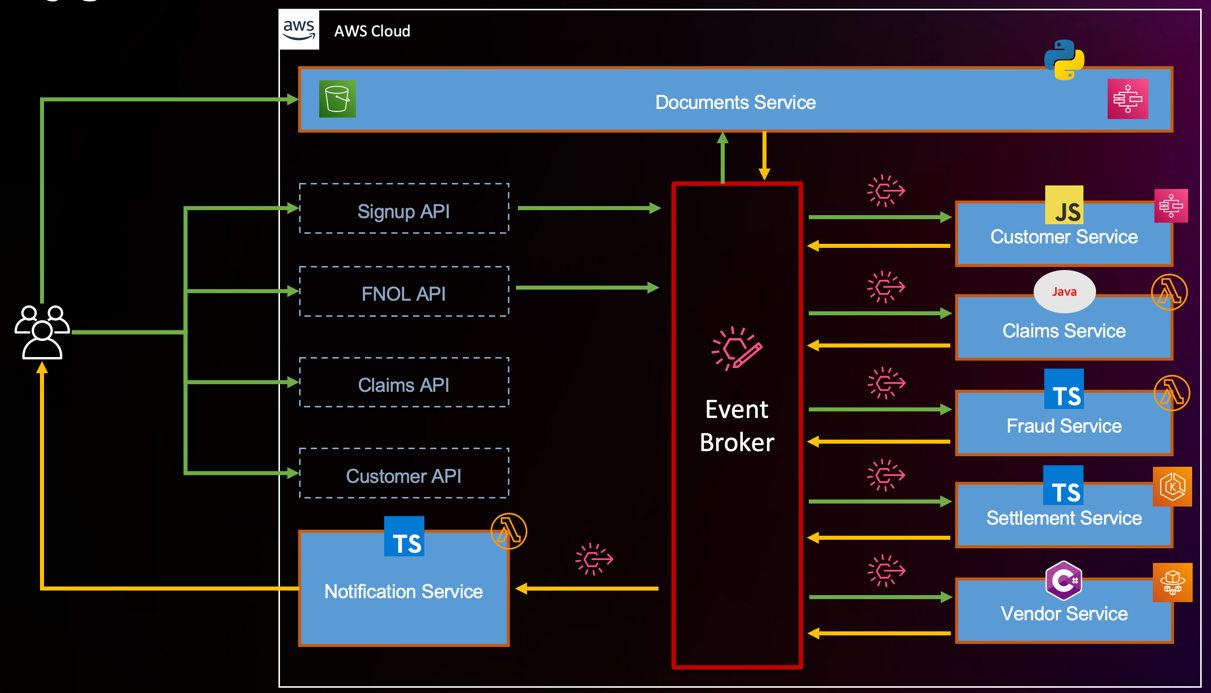
Introduction
.NET Core Web API has emerged as a powerful tool for developing secure, scalable, and high-performance RESTful services. Its cross-platform capabilities make it ideal for integrating modern solutions into diverse systems. In this blog, we’ll explore how a .NET Core Web API was used to streamline insurance claims processing for a client, showcasing the value it can bring to real-world business scenarios.
Client Overview and Challenges
Client Overview
The client, a mid-sized insurance company, processes thousands of claims monthly. They required a robust API to integrate their existing systems, enable third-party access, and provide real-time updates for claim status inquiries.
Challenges
- Manual Claim Processing: Claims were processed manually, leading to delays and errors.
- Disparate Systems: Their internal systems didn’t communicate effectively, creating data silos.
- Lack of Real-Time Updates: Customers couldn’t track their claims in real-time, resulting in frequent support queries.
- Inefficient Third-Party Collaboration: Integrating with third-party systems (e.g., hospitals, repair centers) was cumbersome, leading to delays in claims validation.
The client needed an automated, centralized solution to streamline the claims process and improve customer experience.
Solution: Implementing a Claims Management API with .NET Core Web API
The solution involved building a Claims Management API using .NET Core Web API to automate claim processing, enable seamless third-party integrations, and provide real-time updates.
Key Features of the Solution
1. Automated Claim Submission
- Problem: Manual submissions were error-prone and time-consuming.
- Solution:
- Designed an endpoint (
/api/claims/submit
) to accept claim requests in JSON format. - Validated requests using Data Annotations and Fluent Validation to ensure data integrity.
- Automatically assigned claims to the appropriate department using business logic.
Outcome:
- Reduced claim submission time by 70%, enhancing operational efficiency.
2. Real-Time Claim Status Updates
- Problem: Customers frequently contacted support for claim status updates.
- Solution:
- Built an endpoint (
/api/claims/status/{id}
) to retrieve real-time claim statuses. - Integrated with SignalR to push status updates to customers via email or mobile app notifications.
Outcome:
- Improved customer satisfaction by providing instant updates, reducing support calls by 40%.
3. Third-Party Integrations
- Problem: Collaboration with third-party vendors (e.g., hospitals, garages) was inefficient.
- Solution:
- Created endpoints (
/api/claims/validate
and /api/claims/documents
) for third parties to upload supporting documents and validate claims. - Implemented authentication using JWT (JSON Web Tokens) to ensure secure access.
Outcome:
- Accelerated claim validations, reducing processing time by 50%.
4. Secure and Scalable Architecture
- Problem: The client needed to handle increasing traffic securely.
- Solution:
- Utilized middleware for logging, exception handling, and request validation.
- Secured APIs using OAuth2 and role-based authorization to restrict access.
- Deployed the API on Azure App Services with autoscaling to handle peak loads.
Outcome:
- Achieved 99.9% uptime and ensured compliance with security standards.
5. Comprehensive Reporting
- Problem: The client lacked insights into claims data for decision-making.
- Solution:
- Developed an endpoint (
/api/claims/reports
) to generate real-time reports on claim volumes, processing times, and approvals. - Integrated with Power BI for advanced analytics and visualization.
Outcome:
- Enabled data-driven decision-making, helping the client optimize operations.
Implementation Process
1. Requirement Gathering
- Conducted workshops to understand the client’s pain points and business processes.
- Mapped out the API’s functionality and integration points.
2. API Development
- Followed a modular approach to build reusable controllers and services.
- Used Swagger/OpenAPI for API documentation and testing.
3. Integration
- Connected the API with internal systems (e.g., customer portal, CRM) and third-party platforms.
- Conducted integration testing to ensure smooth data flow.
4. Deployment
- Deployed the API on Azure, leveraging CI/CD pipelines for automated updates.
- Monitored API performance using Application Insights.
Results Achieved
Increased Efficiency
- Automated claim processing reduced manual intervention, saving time and resources.
Enhanced Customer Experience
- Real-time updates and faster claim approvals improved customer satisfaction scores by 25%.
Streamlined Operations
- Seamless third-party integrations eliminated bottlenecks, speeding up claim validations.
Scalability and Reliability
- The scalable architecture handled a 200% increase in traffic without performance issues.
Actionable Insights
- Advanced reporting provided the client with valuable insights to refine their processes.
Sample Use Case: Hospital Claim Validation
Scenario
A customer submitted a medical claim for hospital expenses. Previously, the validation process involved manual coordination between the insurance company and the hospital, causing delays.
.NET Core Web API Solution
- The customer submitted the claim via the
/api/claims/submit
endpoint. - The hospital uploaded supporting documents (e.g., medical bills) through the
/api/claims/documents
endpoint. - The API automatically validated the claim using pre-defined rules and approved it.
- The customer received a real-time notification of the approval via SignalR.
Result:
The claim was processed in under 24 hours, compared to the previous 3-5 days.
Technical Highlights
Tools and Technologies:
- Framework: .NET Core 6.0
- Database: SQL Server with Entity Framework Core
- Authentication: OAuth2 with JWT
- Hosting: Azure App Services
Key Design Patterns:
- Repository Pattern for data access.
- Dependency Injection (DI) for modular and testable code.
- Circuit Breaker Pattern using Polly for resilience.
Performance Optimization:
- Enabled response caching to reduce redundant database calls.
- Used Asynchronous Programming to handle concurrent requests efficiently.