Exploring Local Storage in Angular:
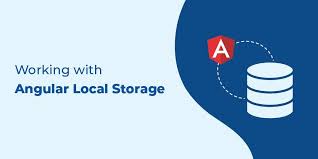
In modern web development, persisting data on the client-side is a crucial aspect, especially in Angular applications where user interactions play a significant role. Local storage is one such mechanism that allows developers to store key-value pairs locally within the user's browser. In this blog post, we'll delve into the world of local storage in Angular, exploring its usage, best practices, and potential pitfalls. Understanding Local Storage
Local storage is a simple key-value storage mechanism available in web browsers. It provides a way to store data persistently across browser sessions. The data stored in local storage remains intact even after the user closes the browser or refreshes the page. Integration with AngularAngular makes it straightforward to work with local storage using TypeScript. Here's a step-by-step guide on how to integrate local storage into your Angular application: 1. Import Local Storage ServiceAngular doesn't have built-in support for local storage, so you'll need to create a service to interact with it. Start by creating a service to encapsulate local storage operations. Typescript:
// local-storage.service.ts import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root'})export class LocalStorageService { constructor() { } setItem(key: string, value: any): void { localStorage.setItem(key, JSON.stringify(value)); } getItem(key: string): any { const item = localStorage.getItem(key); return item ? JSON.parse(item) : null; } removeItem(key: string): void { localStorage.removeItem(key); } clear(): void { localStorage.clear(); }}
2. Using Local Storage in ComponentsOnce the service is set up, you can inject it into your Angular components and use it to store and retrieve data. Typescript:// example.component.ts import { Component, OnInit } from '@angular/core';import { LocalStorageService } from 'path-to-local-storage-service'; @Component({ selector: 'app-example', templateUrl: './example.component.html', styleUrls: ['./example.component.css']})export class ExampleComponent implements OnInit { constructor(private localStorageService: LocalStorageService) { } ngOnInit(): void { // Storing data this.localStorageService.setItem('user', { name: 'John', age: 30 }); // Retrieving data const user = this.localStorageService.getItem('user'); console.log(user); // { name: 'John', age: 30 } }}
3. Best Practices and ConsiderationsWhile local storage is convenient, it's essential to use it judiciously and consider the following best practices: Security: Be cautious about storing sensitive information in local storage as it's accessible by JavaScript running on the same domain.
Storage Limits: Local storage has size limits (typically around 5-10 MB per domain). Avoid storing large amounts of data.
JSON Serialization: Always serialize complex data structures (e.g., objects or arrays) using JSON.stringify() before storing them in local storage and deserialize them using JSON.parse() when retrieving.
ConclusionLocal storage in Angular provides a convenient way to store small amounts of data on the client-side, enabling persistent user experiences. By following best practices and leveraging Angular's capabilities, you can effectively utilize local storage in your applications. Remember to handle data securely and be mindful of storage limits for optimal performance. Happy coding!