C# (CSharp)
Introduction
C#(Pronounced as “C Sharp”) is an object-oriented programming language designed by Anders Hejlsberg and developed by Microsoft in the year 2000. it was designed to be simple, versatile, and highly productive, making it an ideal choice for building a wide range of applications, from desktop software to web applications and even mobile apps. It has been now 20+ years since its inception.
C# Version History and Several Key Points
C# 1.0: Foundations for Managed Code
Releasing Year: January 2002.
History: Microsoft released the first version of C# with Visual Studio 2002. The use of Managed Code was introduced with this version. C# 1.0 was the first language that the developer adopted to build .NET applications.
Core Fundamentals: This version set up the basic tools needed for programming, like classes, structs, interfaces, events, properties, and delegates. These are the fundamental elements that make object-oriented programming possible in C#.
C# 1.2: A Minor Update
Releasing Year: April 2003.
History: Released in April 2003 with .NET Framework 1.1, C# 1.2 was a relatively minor update compared to other versions. It primarily focused on small improvements and bug fixes.
Key Features:
· Automatic Dispose in foreach: When iterating over objects that implement IDisposable, C# 1.2 automatically calls Dispose on the iterator during cleanup, ensuring proper resource management.
· Minor language improvements: Some minor syntax enhancements and clarifications were implemented.
C# 2.0: Embracing Generics and More
Releasing Year: November 2005.
History: C# 2.0, released with .NET Framework 2.0 in 2005, marked a significant evolution of the language compared to its predecessors. It introduced several unprecedented features that transformed how developers wrote code.
Key Features:
· Generics: This was the most impactful addition, allowing developers to write reusable code that could work with different data types without sacrificing performance, Generics revolutionized data structures, algorithms, and code libraries.
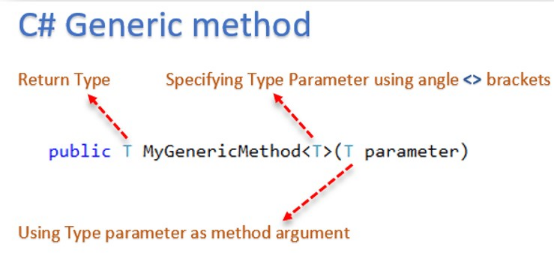
· Partial Classes: Enabled splitting class definitions across multiple files, improving code organization and modularity for large projects.
· Static Classes: Facilitated the creation of classes containing only static members, ideal for utility functions not tied to instances.
· Nullable Types: Enhanced data handling by allowing variables to represent the absence of a value (null). This significantly improved exception handling and code clarity.
· Covariance and Contravariance: Advanced concepts for generic interfaces, enabling more flexible method design in specific scenarios.
C# 3.0: Productivity Boost with LINQ and More
Releasing Year: November 2007.
History: C# 3.0, released in 2007 as part of .NET Framework 3.5, was a game-changer for C# development. It introduced innovative features that significantly enhanced code simplicity, readability, and expressive power.
Key Features:
· Lambda Expression: Concise function definitions within method calls, eliminating boilerplate code and improving readability.
· Extension Method: Extended functionality to existing types dynamically without modifying the original types, promoting loose coupling and maintainability.
· Expression Trees: Enabled creating and manipulating expressions at runtime, opening doors for dynamic code generation and optimization.
· Anonymous Types: Facilitated the creation of temporary lightweight objects without defining separate classes, ideal for small data structures.
· LINQ (Language Integrated Query): Revolutionized data access and manipulation by integrating query expressions directly into the C# language, simplifying common operations like filtering, sorting, and aggregating data.
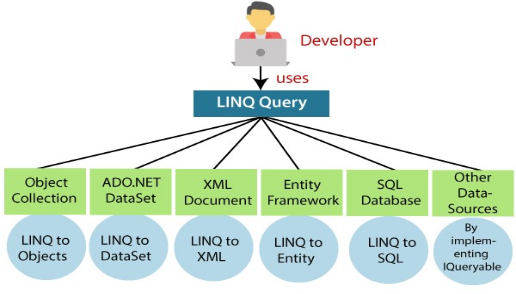
· Implicit Typing (var): Enhanced developer convenience by allowing the compiler to infer variable types, reducing redundant type declarations.
C# 4.0: Refinements and Subtle Enhancements
Releasing Year: April 2010.
History: C# 4.0, released with .NET Framework 4.0 in 2010, focused on refining existing features and enhancing interoperability rather than introducing major paradigm shifts. While not as feature packed as some other versions, it still offered valuable improvements for developers.
Key Features:
· Late Binding: Offered more dynamic behaviour by delaying method resolution until runtime, useful for reflection and introspection.
· Named Arguments Improved readability and maintainability by explicitly specifying argument names in method calls, reducing confusion with positional ordering.
· Optional Parameters: Increased flexibility by allowing methods to accept a variable number of arguments, making them more adaptable to different scenarios.
· Dynamic Keywords: Enabled runtime interaction with dynamic languages like Python or JavaScript, extending C#'s reach beyond statically typed programming.
· More COM Support: Enhanced interoperability with COM components, opening doors for working with existing libraries and technologies.
C# 5.0: Async Programming Enters the Stage
Releasing Year: August 2012.
History: C# version 5.0, released with Visual Studio 2012, was a focused version of the language. The async and await model for asynchronous programming.
Key Features:
· Async Programming: This major addition enabled writing asynchronous code in a more streamlined and readable manner, handling I/O-bound operations without blocking the main thread. This significantly improved responsiveness and performance, especially in applications dealing with network calls, file I/O, or other long-running tasks.
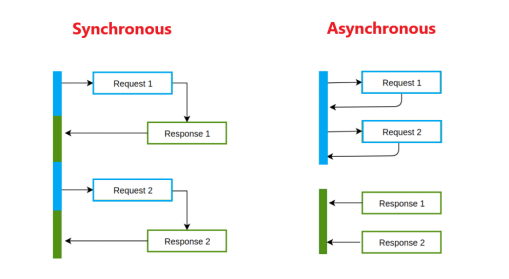
· Caller Information Provided access to metadata about the calling method through attributes, useful for logging, debugging, and other introspection scenarios.